Laravel Telescope
簡介
Laravel Telescope 是您本地 Laravel 開發環境的絕佳夥伴。Telescope 提供了對進入您應用程式的請求、例外、日誌條目、資料庫查詢、佇列任務、郵件、通知、快取操作、排程任務、變數傾印等方面的深入見解。
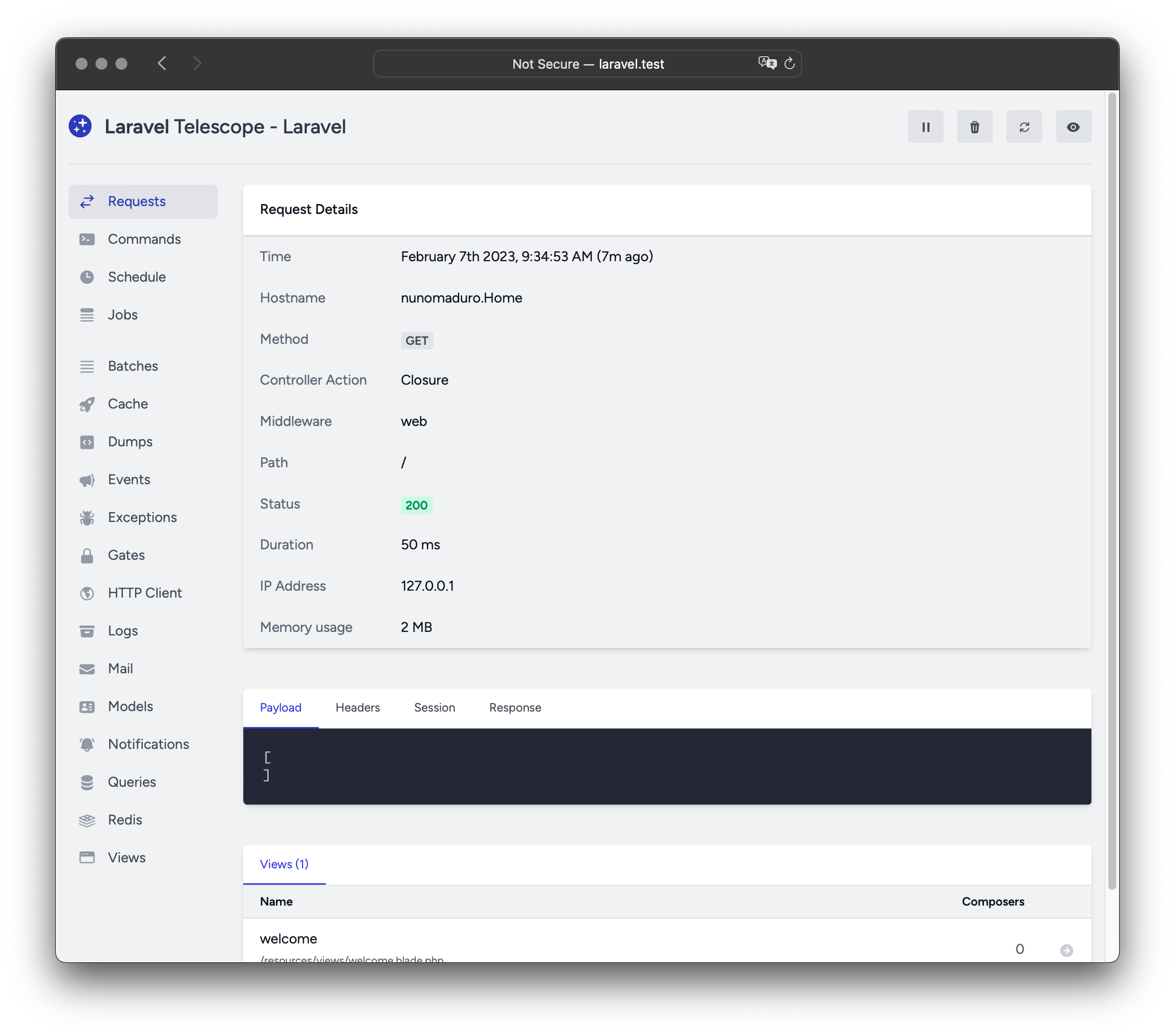
安裝
您可以使用 Composer 套件管理器將 Telescope 安裝到您的 Laravel 專案中
1composer require laravel/telescope
安裝 Telescope 後,使用 telescope:install
Artisan 命令發佈其資源和資料庫遷移。安裝 Telescope 後,您還應該執行 migrate
命令,以建立儲存 Telescope 資料所需的資料表
1php artisan telescope:install2 3php artisan migrate
最後,您可以透過 /telescope
路由存取 Telescope 儀表板。
僅限本地安裝
如果您計劃僅使用 Telescope 來協助本地開發,則可以使用 --dev
標記安裝 Telescope
1composer require laravel/telescope --dev2 3php artisan telescope:install4 5php artisan migrate
執行 telescope:install
後,您應該從應用程式的 bootstrap/providers.php
設定檔中移除 TelescopeServiceProvider
服務提供者的註冊。相反地,請在 App\Providers\AppServiceProvider
類別的 register
方法中手動註冊 Telescope 的服務提供者。我們將確保目前的環境為 local
,然後再註冊提供者
1/** 2 * Register any application services. 3 */ 4public function register(): void 5{ 6 if ($this->app->environment('local') && class_exists(\Laravel\Telescope\TelescopeServiceProvider::class)) { 7 $this->app->register(\Laravel\Telescope\TelescopeServiceProvider::class); 8 $this->app->register(TelescopeServiceProvider::class); 9 }10}
最後,您也應該透過將以下內容新增到您的 composer.json
檔案中,來防止 Telescope 套件被自動探索
1"extra": {2 "laravel": {3 "dont-discover": [4 "laravel/telescope"5 ]6 }7},
設定
發佈 Telescope 的資源後,其主要設定檔將位於 config/telescope.php
。此設定檔可讓您設定watcher 選項。每個設定選項都包含其用途的描述,因此請務必徹底瀏覽此檔案。
如果需要,您可以使用 enabled
設定選項完全停用 Telescope 的資料收集
1'enabled' => env('TELESCOPE_ENABLED', true),
資料修剪
如果沒有修剪,telescope_entries
資料表可能會非常快速地累積記錄。為了減輕這種情況,您應該排程 telescope:prune
Artisan 命令每天執行
1use Illuminate\Support\Facades\Schedule;2 3Schedule::command('telescope:prune')->daily();
預設情況下,所有超過 24 小時的條目都將被修剪。您可以在呼叫命令時使用 hours
選項來決定保留 Telescope 資料的時間長度。例如,以下命令將刪除所有 48 小時前建立的記錄
1use Illuminate\Support\Facades\Schedule;2 3Schedule::command('telescope:prune --hours=48')->daily();
儀表板授權
可以透過 /telescope
路由存取 Telescope 儀表板。預設情況下,您只能在 local
環境中存取此儀表板。在您的 app/Providers/TelescopeServiceProvider.php
檔案中,有一個授權閘道定義。此授權閘道控制對 非本地 環境中 Telescope 的存取。您可以自由地修改此閘道,以根據需要限制對 Telescope 安裝的存取
1use App\Models\User; 2 3/** 4 * Register the Telescope gate. 5 * 6 * This gate determines who can access Telescope in non-local environments. 7 */ 8protected function gate(): void 9{10 Gate::define('viewTelescope', function (User $user) {11 return in_array($user->email, [13 ]);14 });15}
您應確保在您的生產環境中將 APP_ENV
環境變數變更為 production
。否則,您的 Telescope 安裝將公開可用。
升級 Telescope
當升級到 Telescope 的新主要版本時,請務必仔細查看升級指南。
此外,當升級到任何新的 Telescope 版本時,您應該重新發佈 Telescope 的資源
1php artisan telescope:publish
為了保持資源為最新狀態並避免未來更新中出現問題,您可以將 vendor:publish --tag=laravel-assets
命令新增到應用程式 composer.json
檔案中的 post-update-cmd
指令碼
1{2 "scripts": {3 "post-update-cmd": [4 "@php artisan vendor:publish --tag=laravel-assets --ansi --force"5 ]6 }7}
過濾
條目
您可以透過在 App\Providers\TelescopeServiceProvider
類別中定義的 filter
閉包來過濾 Telescope 記錄的資料。預設情況下,此閉包會記錄 local
環境中的所有資料,以及所有其他環境中的例外、失敗任務、排程任務和具有監控標籤的資料
1use Laravel\Telescope\IncomingEntry; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 $this->hideSensitiveRequestDetails();10 11 Telescope::filter(function (IncomingEntry $entry) {12 if ($this->app->environment('local')) {13 return true;14 }15 16 return $entry->isReportableException() ||17 $entry->isFailedJob() ||18 $entry->isScheduledTask() ||19 $entry->isSlowQuery() ||20 $entry->hasMonitoredTag();21 });22}
批次
雖然 filter
閉包會過濾個別條目的資料,但您可以使用 filterBatch
方法註冊一個閉包,以過濾給定請求或命令列命令的所有資料。如果閉包傳回 true
,則 Telescope 會記錄所有條目
1use Illuminate\Support\Collection; 2use Laravel\Telescope\IncomingEntry; 3use Laravel\Telescope\Telescope; 4 5/** 6 * Register any application services. 7 */ 8public function register(): void 9{10 $this->hideSensitiveRequestDetails();11 12 Telescope::filterBatch(function (Collection $entries) {13 if ($this->app->environment('local')) {14 return true;15 }16 17 return $entries->contains(function (IncomingEntry $entry) {18 return $entry->isReportableException() ||19 $entry->isFailedJob() ||20 $entry->isScheduledTask() ||21 $entry->isSlowQuery() ||22 $entry->hasMonitoredTag();23 });24 });25}
標籤
Telescope 允許您依「標籤」搜尋條目。通常,標籤是 Eloquent 模型類別名稱或經過身份驗證的使用者 ID,Telescope 會自動將其新增到條目中。偶爾,您可能想要將自己的自訂標籤附加到條目。若要完成此操作,您可以使用 Telescope::tag
方法。tag
方法接受一個閉包,該閉包應傳回標籤陣列。閉包傳回的標籤將與 Telescope 自動附加到條目的任何標籤合併。通常,您應該在 App\Providers\TelescopeServiceProvider
類別的 register
方法中呼叫 tag
方法
1use Laravel\Telescope\IncomingEntry; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 $this->hideSensitiveRequestDetails();10 11 Telescope::tag(function (IncomingEntry $entry) {12 return $entry->type === 'request'13 ? ['status:'.$entry->content['response_status']]14 : [];15 });16}
可用的 Watchers
當執行請求或命令列命令時,Telescope「watcher」會收集應用程式資料。您可以在 config/telescope.php
設定檔中自訂您想要啟用的 watcher 清單
1'watchers' => [2 Watchers\CacheWatcher::class => true,3 Watchers\CommandWatcher::class => true,4 // ...5],
某些 watcher 也允許您提供額外的自訂選項
1'watchers' => [2 Watchers\QueryWatcher::class => [3 'enabled' => env('TELESCOPE_QUERY_WATCHER', true),4 'slow' => 100,5 ],6 // ...7],
批次 Watcher
批次 watcher 會記錄有關佇列批次的資訊,包括任務和連線資訊。
快取 Watcher
快取 watcher 會在快取鍵被命中、未命中、更新和遺忘時記錄資料。
命令 Watcher
每當執行 Artisan 命令時,命令 watcher 會記錄引數、選項、結束代碼和輸出。如果您想要排除某些命令不被 watcher 記錄,您可以在 config/telescope.php
檔案的 ignore
選項中指定該命令
1'watchers' => [2 Watchers\CommandWatcher::class => [3 'enabled' => env('TELESCOPE_COMMAND_WATCHER', true),4 'ignore' => ['key:generate'],5 ],6 // ...7],
Dump Watcher
Dump watcher 會記錄並在 Telescope 中顯示您的變數傾印。使用 Laravel 時,可以使用全域 dump
函式傾印變數。Dump watcher 索引標籤必須在瀏覽器中開啟才能記錄傾印,否則 watcher 會忽略這些傾印。
事件 Watcher
事件 watcher 會記錄應用程式分派的任何事件的酬載、監聽器和廣播資料。Laravel 框架的內部事件會被事件 watcher 忽略。
例外 Watcher
例外 watcher 會記錄應用程式擲回的任何可報告例外的資料和堆疊追蹤。
Gate Watcher
Gate watcher 會記錄應用程式閘道和策略檢查的資料和結果。如果您想要排除某些能力不被 watcher 記錄,您可以在 config/telescope.php
檔案的 ignore_abilities
選項中指定這些能力
1'watchers' => [2 Watchers\GateWatcher::class => [3 'enabled' => env('TELESCOPE_GATE_WATCHER', true),4 'ignore_abilities' => ['viewNova'],5 ],6 // ...7],
HTTP Client Watcher
HTTP client watcher 會記錄應用程式發出的傳出HTTP client 請求。
任務 Watcher
任務 watcher 會記錄應用程式分派的任何任務的資料和狀態。
日誌 Watcher
日誌 watcher 會記錄應用程式寫入的任何日誌的日誌資料。
預設情況下,Telescope 只會記錄 error
級別或更高級別的日誌。但是,您可以修改應用程式 config/telescope.php
設定檔中的 level
選項來修改此行為
1'watchers' => [2 Watchers\LogWatcher::class => [3 'enabled' => env('TELESCOPE_LOG_WATCHER', true),4 'level' => 'debug',5 ],6 7 // ...8],
郵件 Watcher
郵件 watcher 可讓您檢視應用程式傳送的電子郵件的瀏覽器內預覽,以及其相關資料。您也可以將電子郵件下載為 `.eml` 檔案。
模型 Watcher
每當分派 Eloquent 模型事件時,模型 watcher 就會記錄模型變更。您可以透過 watcher 的 events
選項指定應記錄哪些模型事件
1'watchers' => [2 Watchers\ModelWatcher::class => [3 'enabled' => env('TELESCOPE_MODEL_WATCHER', true),4 'events' => ['eloquent.created*', 'eloquent.updated*'],5 ],6 // ...7],
如果您想要記錄在特定請求期間水合模型的數量,請啟用 hydrations
選項
1'watchers' => [2 Watchers\ModelWatcher::class => [3 'enabled' => env('TELESCOPE_MODEL_WATCHER', true),4 'events' => ['eloquent.created*', 'eloquent.updated*'],5 'hydrations' => true,6 ],7 // ...8],
通知 Watcher
通知監看器會記錄您的應用程式發送的所有通知。如果通知觸發電子郵件,並且您已啟用郵件監看器,則電子郵件也將在郵件監看器螢幕上提供預覽。
查詢 Watcher
查詢監看器會記錄您的應用程式執行的所有查詢的原始 SQL、綁定和執行時間。監看器還會將任何速度慢於 100 毫秒的查詢標記為 slow
。您可以使用監看器的 slow
選項自訂慢查詢閾值
1'watchers' => [2 Watchers\QueryWatcher::class => [3 'enabled' => env('TELESCOPE_QUERY_WATCHER', true),4 'slow' => 50,5 ],6 // ...7],
Redis Watcher
Redis 監看器會記錄您的應用程式執行的所有 Redis 命令。如果您使用 Redis 進行快取,快取命令也將由 Redis 監看器記錄。
請求 Watcher
請求監看器會記錄與應用程式處理的任何請求相關聯的請求、標頭、會話和回應資料。您可以通過 size_limit
(以千位元組為單位)選項限制您記錄的回應資料
1'watchers' => [2 Watchers\RequestWatcher::class => [3 'enabled' => env('TELESCOPE_REQUEST_WATCHER', true),4 'size_limit' => env('TELESCOPE_RESPONSE_SIZE_LIMIT', 64),5 ],6 // ...7],
排程 Watcher
排程監看器會記錄您的應用程式運行的任何排程任務的命令和輸出。
視圖 Watcher
視圖監看器會記錄呈現視圖時使用的視圖名稱、路徑、資料和「composers」。
顯示使用者頭像
Telescope 儀表板會顯示在儲存給定條目時已驗證使用者的使用者頭像。 預設情況下,Telescope 將使用 Gravatar 網路服務檢索頭像。 但是,您可以通過在您的 App\Providers\TelescopeServiceProvider
類別中註冊回呼來自訂頭像 URL。 回呼將接收使用者的 ID 和電子郵件地址,並應傳回使用者的頭像圖片 URL
1use App\Models\User; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 // ...10 11 Telescope::avatar(function (string $id, string $email) {12 return '/avatars/'.User::find($id)->avatar_path;13 });14}