Prompts
簡介
Laravel Prompts 是一個 PHP 套件,用於為您的命令列應用程式新增美觀且使用者友善的表單,並具有類似瀏覽器的功能,包括佔位符文字和驗證。
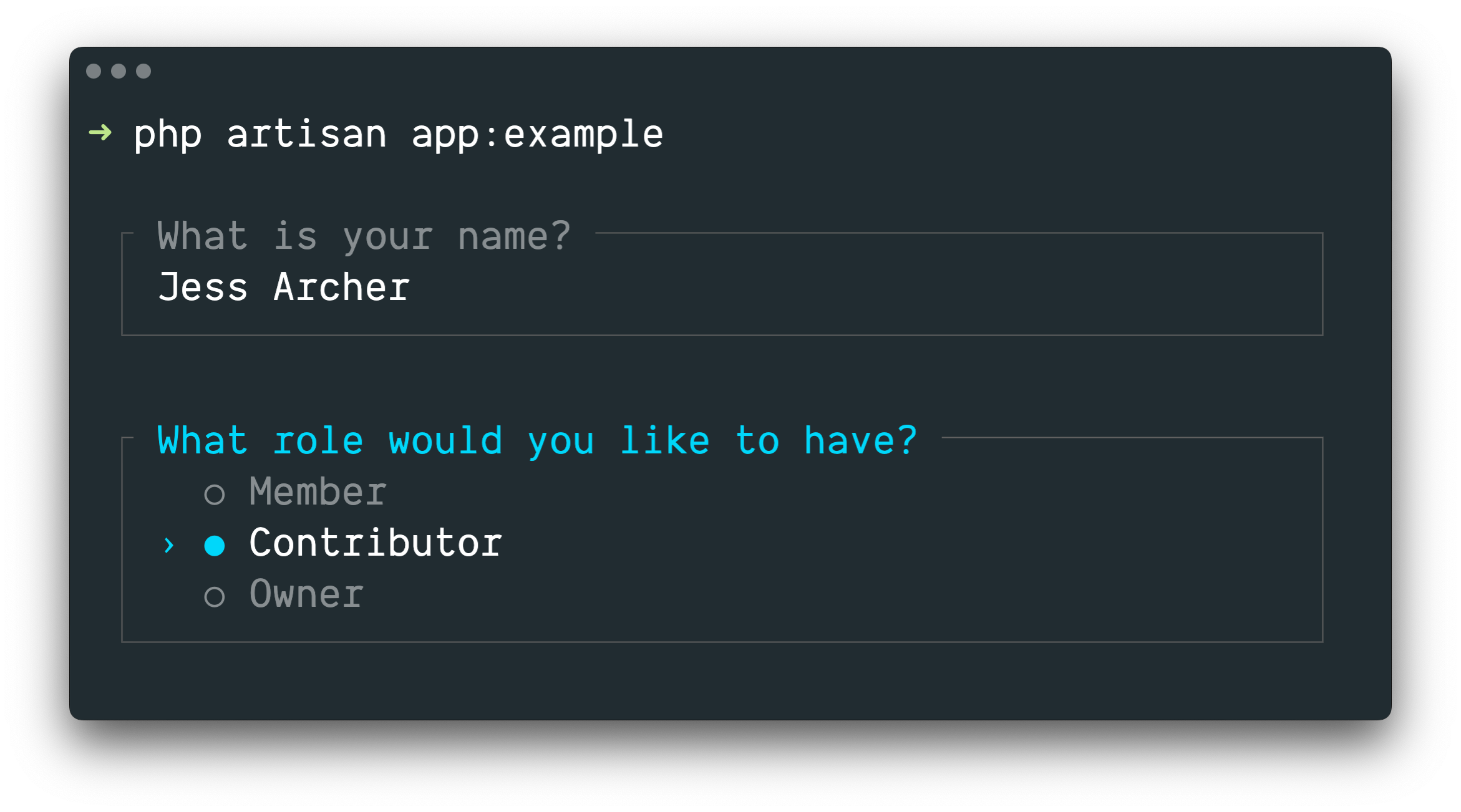
Laravel Prompts 非常適合在您的 Artisan 命令列指令 中接受使用者輸入,但它也可以用於任何命令列 PHP 專案。
Laravel Prompts 支援 macOS、Linux 和 Windows 與 WSL。 如需更多資訊,請參閱我們關於不支援的環境與回退的文件。
安裝
Laravel Prompts 已包含在 Laravel 的最新版本中。
Laravel Prompts 也可以使用 Composer 套件管理器安裝在您的其他 PHP 專案中
1composer require laravel/prompts
可用的 Prompts
文字
text
函式將使用給定的問題提示使用者,接受他們的輸入,然後傳回它
1use function Laravel\Prompts\text;2 3$name = text('What is your name?');
您還可以包含佔位符文字、預設值和資訊提示
1$name = text(2 label: 'What is your name?',3 placeholder: 'E.g. Taylor Otwell',4 default: $user?->name,5 hint: 'This will be displayed on your profile.'6);
必填值
如果您要求必須輸入值,您可以傳遞 required
參數
1$name = text(2 label: 'What is your name?',3 required: true4);
如果您想要自訂驗證訊息,您也可以傳遞字串
1$name = text(2 label: 'What is your name?',3 required: 'Your name is required.'4);
額外驗證
最後,如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$name = text(2 label: 'What is your name?',3 validate: fn (string $value) => match (true) {4 strlen($value) < 3 => 'The name must be at least 3 characters.',5 strlen($value) > 255 => 'The name must not exceed 255 characters.',6 default => null7 }8);
閉包將接收已輸入的值,並可能傳回錯誤訊息,如果驗證通過,則傳回 null
。
或者,您可以利用 Laravel 的 驗證器 的強大功能。 若要這麼做,請提供一個陣列,其中包含屬性名稱和所需的驗證規則給 validate
參數
1$name = text(2 label: 'What is your name?',3 validate: ['name' => 'required|max:255|unique:users']4);
文字區域
textarea
函式將使用給定的問題提示使用者,透過多行文字區域接受他們的輸入,然後傳回它
1use function Laravel\Prompts\textarea;2 3$story = textarea('Tell me a story.');
您還可以包含佔位符文字、預設值和資訊提示
1$story = textarea(2 label: 'Tell me a story.',3 placeholder: 'This is a story about...',4 hint: 'This will be displayed on your profile.'5);
必填值
如果您要求必須輸入值,您可以傳遞 required
參數
1$story = textarea(2 label: 'Tell me a story.',3 required: true4);
如果您想要自訂驗證訊息,您也可以傳遞字串
1$story = textarea(2 label: 'Tell me a story.',3 required: 'A story is required.'4);
額外驗證
最後,如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$story = textarea(2 label: 'Tell me a story.',3 validate: fn (string $value) => match (true) {4 strlen($value) < 250 => 'The story must be at least 250 characters.',5 strlen($value) > 10000 => 'The story must not exceed 10,000 characters.',6 default => null7 }8);
閉包將接收已輸入的值,並可能傳回錯誤訊息,如果驗證通過,則傳回 null
。
或者,您可以利用 Laravel 的 驗證器 的強大功能。 若要這麼做,請提供一個陣列,其中包含屬性名稱和所需的驗證規則給 validate
參數
1$story = textarea(2 label: 'Tell me a story.',3 validate: ['story' => 'required|max:10000']4);
密碼
password
函式與 text
函式類似,但當使用者在命令列中輸入時,他們的輸入將被遮罩。 這在詢問敏感資訊(例如密碼)時很有用
1use function Laravel\Prompts\password;2 3$password = password('What is your password?');
您還可以包含佔位符文字和資訊提示
1$password = password(2 label: 'What is your password?',3 placeholder: 'password',4 hint: 'Minimum 8 characters.'5);
必填值
如果您要求必須輸入值,您可以傳遞 required
參數
1$password = password(2 label: 'What is your password?',3 required: true4);
如果您想要自訂驗證訊息,您也可以傳遞字串
1$password = password(2 label: 'What is your password?',3 required: 'The password is required.'4);
額外驗證
最後,如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$password = password(2 label: 'What is your password?',3 validate: fn (string $value) => match (true) {4 strlen($value) < 8 => 'The password must be at least 8 characters.',5 default => null6 }7);
閉包將接收已輸入的值,並可能傳回錯誤訊息,如果驗證通過,則傳回 null
。
或者,您可以利用 Laravel 的 驗證器 的強大功能。 若要這麼做,請提供一個陣列,其中包含屬性名稱和所需的驗證規則給 validate
參數
1$password = password(2 label: 'What is your password?',3 validate: ['password' => 'min:8']4);
確認
如果您需要詢問使用者「是或否」的確認,您可以使用 confirm
函式。 使用者可以使用方向鍵或按下 y
或 n
來選擇他們的回應。 此函式將傳回 true
或 false
。
1use function Laravel\Prompts\confirm;2 3$confirmed = confirm('Do you accept the terms?');
您還可以包含預設值、自訂「是」和「否」標籤的措辭,以及資訊提示
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 default: false,4 yes: 'I accept',5 no: 'I decline',6 hint: 'The terms must be accepted to continue.'7);
要求「是」
如有必要,您可以透過傳遞 required
參數來要求使用者選擇「是」
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 required: true4);
如果您想要自訂驗證訊息,您也可以傳遞字串
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 required: 'You must accept the terms to continue.'4);
選擇
如果您需要使用者從預定義的選項集中選擇,您可以使用 select
函式
1use function Laravel\Prompts\select;2 3$role = select(4 label: 'What role should the user have?',5 options: ['Member', 'Contributor', 'Owner']6);
您還可以指定預設選項和資訊提示
1$role = select(2 label: 'What role should the user have?',3 options: ['Member', 'Contributor', 'Owner'],4 default: 'Owner',5 hint: 'The role may be changed at any time.'6);
您還可以將關聯陣列傳遞給 options
參數,以傳回選定的鍵而不是其值
1$role = select(2 label: 'What role should the user have?',3 options: [4 'member' => 'Member',5 'contributor' => 'Contributor',6 'owner' => 'Owner',7 ],8 default: 'owner'9);
在列表開始滾動之前,最多會顯示五個選項。 您可以透過傳遞 scroll
參數來自訂此設定
1$role = select(2 label: 'Which category would you like to assign?',3 options: Category::pluck('name', 'id'),4 scroll: 105);
額外驗證
與其他提示函式不同,select
函式不接受 required
參數,因為不可能不選擇任何選項。 但是,如果您需要呈現一個選項但阻止其被選取,您可以將閉包傳遞給 validate
參數
1$role = select( 2 label: 'What role should the user have?', 3 options: [ 4 'member' => 'Member', 5 'contributor' => 'Contributor', 6 'owner' => 'Owner', 7 ], 8 validate: fn (string $value) => 9 $value === 'owner' && User::where('role', 'owner')->exists()10 ? 'An owner already exists.'11 : null12);
如果 options
參數是關聯陣列,則閉包將接收選定的鍵,否則它將接收選定的值。 閉包可能會傳回錯誤訊息,如果驗證通過,則傳回 null
。
多選
如果您需要使用者能夠選擇多個選項,您可以使用 multiselect
函式
1use function Laravel\Prompts\multiselect;2 3$permissions = multiselect(4 label: 'What permissions should be assigned?',5 options: ['Read', 'Create', 'Update', 'Delete']6);
您還可以指定預設選項和資訊提示
1use function Laravel\Prompts\multiselect;2 3$permissions = multiselect(4 label: 'What permissions should be assigned?',5 options: ['Read', 'Create', 'Update', 'Delete'],6 default: ['Read', 'Create'],7 hint: 'Permissions may be updated at any time.'8);
您還可以將關聯陣列傳遞給 options
參數,以傳回選定選項的鍵而不是它們的值
1$permissions = multiselect( 2 label: 'What permissions should be assigned?', 3 options: [ 4 'read' => 'Read', 5 'create' => 'Create', 6 'update' => 'Update', 7 'delete' => 'Delete', 8 ], 9 default: ['read', 'create']10);
在列表開始滾動之前,最多會顯示五個選項。 您可以透過傳遞 scroll
參數來自訂此設定
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 scroll: 105);
要求值
預設情況下,使用者可以選擇零個或多個選項。 您可以傳遞 required
參數以強制要求一個或多個選項
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 required: true5);
如果您想要自訂驗證訊息,您可以將字串提供給 required
參數
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 required: 'You must select at least one category'5);
額外驗證
如果您需要呈現一個選項但阻止其被選取,您可以將閉包傳遞給 validate
參數
1$permissions = multiselect( 2 label: 'What permissions should the user have?', 3 options: [ 4 'read' => 'Read', 5 'create' => 'Create', 6 'update' => 'Update', 7 'delete' => 'Delete', 8 ], 9 validate: fn (array $values) => ! in_array('read', $values)10 ? 'All users require the read permission.'11 : null12);
如果 options
參數是關聯陣列,則閉包將接收選定的鍵,否則它將接收選定的值。 閉包可能會傳回錯誤訊息,如果驗證通過,則傳回 null
。
建議
suggest
函式可用於為可能的選項提供自動完成功能。 使用者仍然可以提供任何答案,無論自動完成提示如何
1use function Laravel\Prompts\suggest;2 3$name = suggest('What is your name?', ['Taylor', 'Dayle']);
或者,您可以將閉包作為第二個參數傳遞給 suggest
函式。 每次使用者輸入字元時都會呼叫閉包。 閉包應接受一個字串參數,其中包含使用者到目前為止的輸入,並傳回自動完成的選項陣列
1$name = suggest(2 label: 'What is your name?',3 options: fn ($value) => collect(['Taylor', 'Dayle'])4 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))5)
您還可以包含佔位符文字、預設值和資訊提示
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 placeholder: 'E.g. Taylor',5 default: $user?->name,6 hint: 'This will be displayed on your profile.'7);
必填值
如果您要求必須輸入值,您可以傳遞 required
參數
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 required: true5);
如果您想要自訂驗證訊息,您也可以傳遞字串
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 required: 'Your name is required.'5);
額外驗證
最後,如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 validate: fn (string $value) => match (true) {5 strlen($value) < 3 => 'The name must be at least 3 characters.',6 strlen($value) > 255 => 'The name must not exceed 255 characters.',7 default => null8 }9);
閉包將接收已輸入的值,並可能傳回錯誤訊息,如果驗證通過,則傳回 null
。
或者,您可以利用 Laravel 的 驗證器 的強大功能。 若要這麼做,請提供一個陣列,其中包含屬性名稱和所需的驗證規則給 validate
參數
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 validate: ['name' => 'required|min:3|max:255']5);
搜尋
如果您有很多選項供使用者選擇,則 search
函式允許使用者輸入搜尋查詢以篩選結果,然後使用方向鍵選擇選項
1use function Laravel\Prompts\search;2 3$id = search(4 label: 'Search for the user that should receive the mail',5 options: fn (string $value) => strlen($value) > 06 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()7 : []8);
閉包將接收使用者到目前為止輸入的文字,並且必須傳回選項陣列。 如果您傳回關聯陣列,則將傳回選定選項的鍵,否則將傳回其值。
當篩選您打算傳回值的陣列時,您應該使用 array_values
函式或 values
Collection 方法,以確保陣列不會變成關聯陣列
1$names = collect(['Taylor', 'Abigail']);2 3$selected = search(4 label: 'Search for the user that should receive the mail',5 options: fn (string $value) => $names6 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))7 ->values()8 ->all(),9);
您還可以包含佔位符文字和資訊提示
1$id = search(2 label: 'Search for the user that should receive the mail',3 placeholder: 'E.g. Taylor Otwell',4 options: fn (string $value) => strlen($value) > 05 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()6 : [],7 hint: 'The user will receive an email immediately.'8);
在列表開始滾動之前,最多會顯示五個選項。 您可以透過傳遞 scroll
參數來自訂此設定
1$id = search(2 label: 'Search for the user that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 scroll: 107);
額外驗證
如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$id = search( 2 label: 'Search for the user that should receive the mail', 3 options: fn (string $value) => strlen($value) > 0 4 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all() 5 : [], 6 validate: function (int|string $value) { 7 $user = User::findOrFail($value); 8 9 if ($user->opted_out) {10 return 'This user has opted-out of receiving mail.';11 }12 }13);
如果 options
閉包傳回關聯陣列,則閉包將接收選定的鍵,否則,它將接收選定的值。 閉包可能會傳回錯誤訊息,如果驗證通過,則傳回 null
。
多重搜尋
如果您有很多可搜尋的選項,並且需要使用者能夠選擇多個項目,則 multisearch
函式允許使用者輸入搜尋查詢以篩選結果,然後使用方向鍵和空白鍵選擇選項
1use function Laravel\Prompts\multisearch;2 3$ids = multisearch(4 'Search for the users that should receive the mail',5 fn (string $value) => strlen($value) > 06 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()7 : []8);
閉包將接收使用者到目前為止輸入的文字,並且必須傳回選項陣列。 如果您傳回關聯陣列,則將傳回選定選項的鍵;否則,將傳回它們的值。
當篩選您打算傳回值的陣列時,您應該使用 array_values
函式或 values
Collection 方法,以確保陣列不會變成關聯陣列
1$names = collect(['Taylor', 'Abigail']);2 3$selected = multisearch(4 label: 'Search for the users that should receive the mail',5 options: fn (string $value) => $names6 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))7 ->values()8 ->all(),9);
您還可以包含佔位符文字和資訊提示
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 placeholder: 'E.g. Taylor Otwell',4 options: fn (string $value) => strlen($value) > 05 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()6 : [],7 hint: 'The user will receive an email immediately.'8);
在列表開始滾動之前,最多會顯示五個選項。 您可以透過提供 scroll
參數來自訂此設定
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 scroll: 107);
要求值
預設情況下,使用者可以選擇零個或多個選項。 您可以傳遞 required
參數以強制要求一個或多個選項
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 required: true7);
如果您想要自訂驗證訊息,您也可以將字串提供給 required
參數
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 required: 'You must select at least one user.'7);
額外驗證
如果您想要執行額外的驗證邏輯,您可以將閉包傳遞給 validate
參數
1$ids = multisearch( 2 label: 'Search for the users that should receive the mail', 3 options: fn (string $value) => strlen($value) > 0 4 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all() 5 : [], 6 validate: function (array $values) { 7 $optedOut = User::whereLike('name', '%a%')->findMany($values); 8 9 if ($optedOut->isNotEmpty()) {10 return $optedOut->pluck('name')->join(', ', ', and ').' have opted out.';11 }12 }13);
如果 options
閉包傳回關聯陣列,則閉包將接收選定的鍵;否則,它將接收選定的值。 閉包可能會傳回錯誤訊息,如果驗證通過,則傳回 null
。
暫停
pause
函式可用於向使用者顯示資訊文字,並等待他們按下 Enter / Return 鍵以確認他們想要繼續
1use function Laravel\Prompts\pause;2 3pause('Press ENTER to continue.');
驗證前轉換輸入
有時您可能希望在驗證發生之前轉換提示輸入。 例如,您可能希望從任何提供的字串中刪除空白字元。 為了實現這一點,許多提示函式都提供了 transform
參數,它接受閉包
1$name = text(2 label: 'What is your name?',3 transform: fn (string $value) => trim($value),4 validate: fn (string $value) => match (true) {5 strlen($value) < 3 => 'The name must be at least 3 characters.',6 strlen($value) > 255 => 'The name must not exceed 255 characters.',7 default => null8 }9);
表單
通常,您將有多個提示將按順序顯示,以收集資訊,然後再執行其他動作。 您可以使用 form
函式來建立一組分組的提示,供使用者完成
1use function Laravel\Prompts\form;2 3$responses = form()4 ->text('What is your name?', required: true)5 ->password('What is your password?', validate: ['password' => 'min:8'])6 ->confirm('Do you accept the terms?')7 ->submit();
submit
方法將傳回一個數字索引的陣列,其中包含來自表單提示的所有回應。 但是,您可以透過 name
參數為每個提示提供名稱。 當提供名稱時,可以透過該名稱存取已命名的提示的回應
1use App\Models\User; 2use function Laravel\Prompts\form; 3 4$responses = form() 5 ->text('What is your name?', required: true, name: 'name') 6 ->password( 7 label: 'What is your password?', 8 validate: ['password' => 'min:8'], 9 name: 'password'10 )11 ->confirm('Do you accept the terms?')12 ->submit();13 14User::create([15 'name' => $responses['name'],16 'password' => $responses['password'],17]);
使用 form
函式的主要好處是使用者可以使用 CTRL + U
返回表單中的先前提示。 這允許使用者修正錯誤或變更選擇,而無需取消並重新啟動整個表單。
如果您需要對表單中的提示進行更精細的控制,您可以調用 add
方法,而不是直接呼叫其中一個提示函式。 add
方法會傳遞使用者提供的所有先前回應
1use function Laravel\Prompts\form; 2use function Laravel\Prompts\outro; 3 4$responses = form() 5 ->text('What is your name?', required: true, name: 'name') 6 ->add(function ($responses) { 7 return text("How old are you, {$responses['name']}?"); 8 }, name: 'age') 9 ->submit();10 11outro("Your name is {$responses['name']} and you are {$responses['age']} years old.");
資訊訊息
note
、info
、warning
、error
和 alert
函式可用於顯示資訊訊息
1use function Laravel\Prompts\info;2 3info('Package installed successfully.');
表格
table
函式可以輕鬆顯示多行和多列資料。 您只需要提供表格的欄名稱和資料
1use function Laravel\Prompts\table;2 3table(4 headers: ['Name', 'Email'],5 rows: User::all(['name', 'email'])->toArray()6);
旋轉
spin
函式在執行指定的迴呼時,會顯示旋轉符號以及可選訊息。 它用於指示正在進行的程序,並在完成後傳回迴呼的結果
1use function Laravel\Prompts\spin;2 3$response = spin(4 message: 'Fetching response...',5 callback: fn () => Http::get('http://example.com')6);
spin
函式需要 pcntl
PHP 擴充功能來動畫旋轉符號。 當此擴充功能不可用時,將會改為顯示旋轉符號的靜態版本。
進度條
對於長時間運行的任務,顯示進度條以告知使用者任務的完成進度可能會很有幫助。 使用 progress
函式,Laravel 將顯示進度條,並針對給定可迭代值的每次迭代推進其進度
1use function Laravel\Prompts\progress;2 3$users = progress(4 label: 'Updating users',5 steps: User::all(),6 callback: fn ($user) => $this->performTask($user)7);
progress
函式的行為類似於 map 函式,並將傳回一個陣列,其中包含迴呼的每次迭代的傳回值。
迴呼也可以接受 Laravel\Prompts\Progress
實例,允許您在每次迭代時修改標籤和提示
1$users = progress( 2 label: 'Updating users', 3 steps: User::all(), 4 callback: function ($user, $progress) { 5 $progress 6 ->label("Updating {$user->name}") 7 ->hint("Created on {$user->created_at}"); 8 9 return $this->performTask($user);10 },11 hint: 'This may take some time.'12);
有時,您可能需要更手動地控制進度條的推進方式。 首先,定義程序將迭代的總步數。 然後,在處理完每個項目後,透過 advance
方法推進進度條
1$progress = progress(label: 'Updating users', steps: 10); 2 3$users = User::all(); 4 5$progress->start(); 6 7foreach ($users as $user) { 8 $this->performTask($user); 9 10 $progress->advance();11}12 13$progress->finish();
清除終端機
clear
函式可用於清除使用者的終端機
1use function Laravel\Prompts\clear;2 3clear();
終端機考量
終端機寬度
如果任何標籤、選項或驗證訊息的長度超過使用者終端機中的「欄」數,則會自動截斷以適合。 如果您的使用者可能正在使用較窄的終端機,請考慮盡量縮短這些字串的長度。 通常安全的最大長度為 74 個字元,以支援 80 個字元的終端機。
終端機高度
對於任何接受 scroll
參數的提示,設定的值將自動減少以適合使用者終端機的高度,包括驗證訊息的空間。
不支援的環境與回退
Laravel Prompts 支援 macOS、Linux 和 Windows 與 WSL。 由於 Windows 版本 PHP 的限制,目前無法在 WSL 之外的 Windows 上使用 Laravel Prompts。
因此,Laravel Prompts 支援回退到替代實作,例如 Symfony Console Question Helper。
當將 Laravel Prompts 與 Laravel 框架一起使用時,已為您設定了每個提示的回退,並且將在不支援的環境中自動啟用。
回退條件
如果您未使用 Laravel 或需要自訂何時使用回退行為,您可以將布林值傳遞給 Prompt
類別上的 fallbackWhen
靜態方法
1use Laravel\Prompts\Prompt;2 3Prompt::fallbackWhen(4 ! $input->isInteractive() || windows_os() || app()->runningUnitTests()5);
回退行為
如果您未使用 Laravel 或需要自訂回退行為,您可以將閉包傳遞給每個提示類別上的 fallbackUsing
靜態方法
1use Laravel\Prompts\TextPrompt; 2use Symfony\Component\Console\Question\Question; 3use Symfony\Component\Console\Style\SymfonyStyle; 4 5TextPrompt::fallbackUsing(function (TextPrompt $prompt) use ($input, $output) { 6 $question = (new Question($prompt->label, $prompt->default ?: null)) 7 ->setValidator(function ($answer) use ($prompt) { 8 if ($prompt->required && $answer === null) { 9 throw new \RuntimeException(10 is_string($prompt->required) ? $prompt->required : 'Required.'11 );12 }13 14 if ($prompt->validate) {15 $error = ($prompt->validate)($answer ?? '');16 17 if ($error) {18 throw new \RuntimeException($error);19 }20 }21 22 return $answer;23 });24 25 return (new SymfonyStyle($input, $output))26 ->askQuestion($question);27});
必須為每個提示類別單獨設定回退。 閉包將接收提示類別的實例,並且必須傳回提示的適當類型。